Overview
OpenID Connect—or OIDC—is an identity layer. It lets you build support for signing into your application via the user’s email verified Dropbox identity, which reduces the number of steps for a user to sign up for your service.
This provides the end user with a simple consent screen, and returns basic profile information to your application.
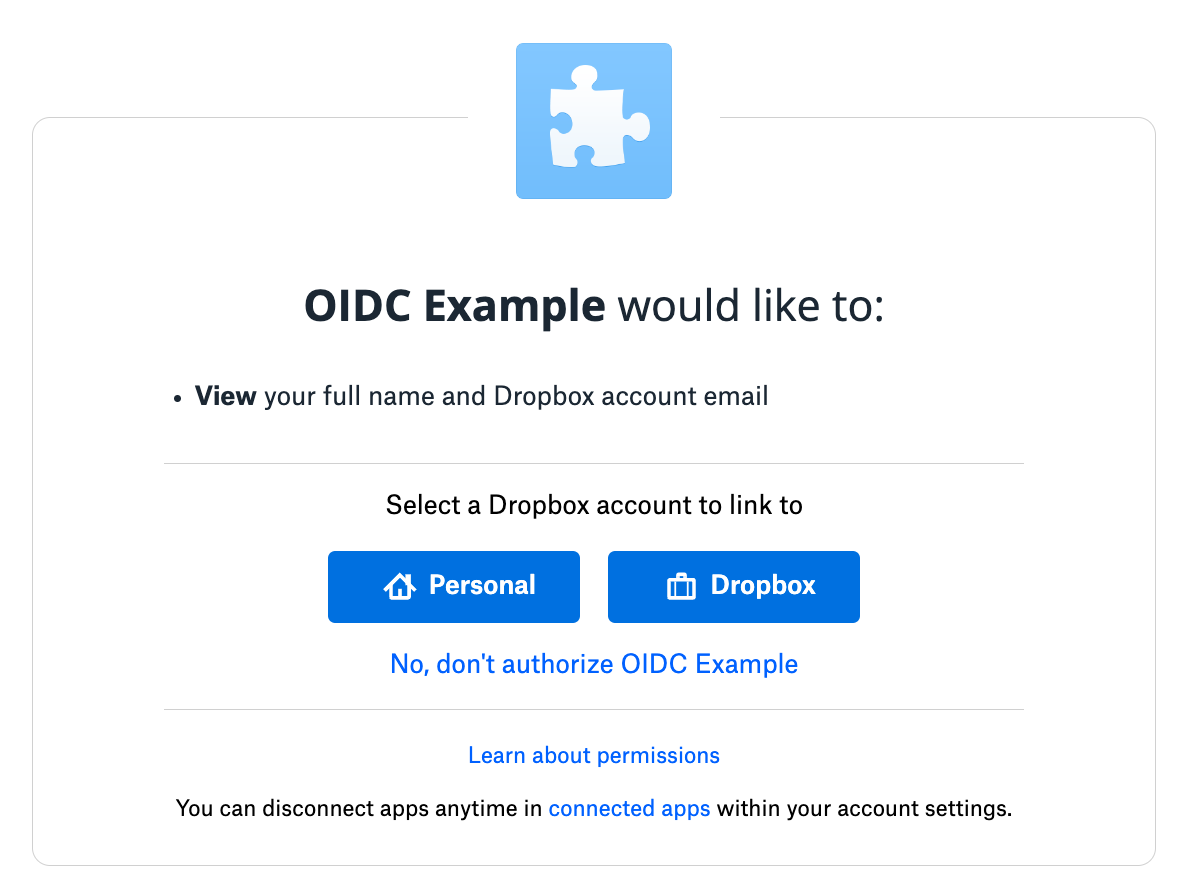
OIDC is built on top of OAuth 2.0 so be sure to read our OAuth Guide before proceeding. The remainder of the guide assumes familiarity with the OAuth code flow and scopes.
Implementing OIDC
Scope Selection
To use OIDC in your request, be sure to select the openid scope and at least one of profile or email. The profile scope is required to view first and last name, and email to view email.
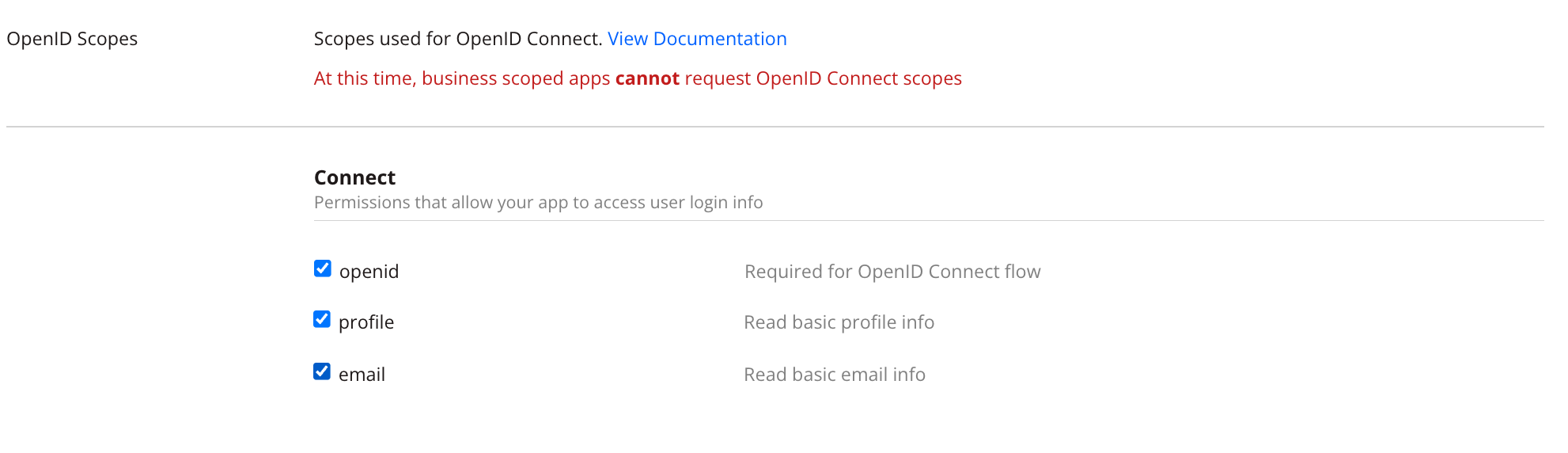
OpenID Connect scopes in the Permissions tab in the app settings for a Dropbox app
Authorization URL
To use OIDC, be sure to specify them in the scope parameter of your /oauth2/authorize authorization URL:
https://www.dropbox.com/oauth2/authorize?client_id=YOUR_CLIENT_ID&response_type=code&scope=openid email profile
Note that the OIDC scopes must be explicitly requested via the scope parameter—they will not be included by default if not included in the scope parameter.
OIDC is only supported when using the code grant, that is, with response_type=code.
Retrieving Identity
When OIDC scopes are requested, the result of the /oauth2/token call will contain the return value id_token in addition to its other return types. The id_token is a JSON Web Token which is a series of three character strings separated by periods: header, payload, and signature. They can be parsed with standard JWT libraries; header and payload are base64-encoded.
The parsed JWT will contain identity information in this format:
{
"email":"your-email@domain.com",
"email_verified":true,
"family_name":"Ferdinand",
"given_name":"Franz",
"iss":"https://www.dropbox.com",
"sub":"dbid:AAH4f99T0taONIb-OurWxbNQ6ywGRopQngc",
"exp":1655080600,
"iat":1655077000,
"aud":"a12fbpq596acfbc",
"auth_time":1655077000,
"dropbox:team_id":"dbtid:WxbNQOurtaONIb-AAH4fQngc99T06ywGRop"
}
Alternatively, the identity information may be retrieved via the /openid/userinfo endpoint.
Email verification
The OIDC flow guarantees email verification—email_verified will always return true. If the user has not verified their email address on Dropbox, they will be prompted to do so during the OAuth app authorization flow when requesting OIDC scopes.
Email verification is not required for non-OIDC authorization flows. To verify identity, use OIDC.
OIDC Standard
OIDC is an open standard. It is supported by many tools and libraries which can simplify your implementation. Dropbox has implemented mandatory parts of the specification.
Discovery Documents
Dropbox has published standard discovery documents:
- https://www.dropbox.com/.well-known/openid-configuration describes supported parameters and options.
- https://www.dropbox.com/.well-known/jwks contains public verification keys in JWK format for verifying signed ID tokens.
Claims
Dropbox’s OIDC implementation supports the following claims:
Claim | Description |
---|---|
iss | The Issuer Identifier (always https://www.dropbox.com). |
sub | An identifier for the user. This is the Dropbox account_id, a string value such as dbid:AAH4f99T0taONIb-OurWxbNQ6ywGRopQngc. |
aud | The audience this ID token is intend for. This will be your application’s client_id. |
iat | The time the ID token was issued, as a Unix timestamp. |
exp | The time the ID token expires, as a Unix timestamp. |
family_name | Last name |
given_name | First name |
Email address | |
email_verified | If the email address has been verified (always true). |
Authorization URL
Dropbox support mandatory parameters specified by OIDC on the authorization URL.
The prompt consent and login parameter values are aliases of the force_reapprove and force_reauthentication parameters, respectively.
The OIDC display, preferred_locales, and acr_values are accepted for compatibility but are non-operational.
Summary
OIDC makes it easier to incorporate Sign in with Dropbox functionality into your applications.
The feature is now available as developer preview. As OIDC is a standard we do not envision incompatible changes, and consider the functionality safe to use in production environments. SDK coverage of OIDC functionality is limited; the functionality will be marked fully supported upon larger SDK coverage.