[November 10, 2023 Update: We’ve updated this Team Files Guide to incorporate information about new features and changes to the Dropbox API around accessing team content. You can find more information about these updates in this blog post.]
Introduction
Dropbox for teams brought the power of Dropbox to organizations and teams. Working with team content is slightly different than managing personal content. This document will guide you through the many capabilities of the Dropbox API to manipulate team content.
If you are relatively new to the Dropbox API, we strongly recommend going through the File Access Guide and the Sharing Guide prior to this guide.
There are specific endpoints to interact with team features, and some important things to know when accessing team member files. In this guide we are going to cover the main API operations that involve team files and related concepts such as namespaces, Select-User, Select-Admin, and Path-Root headers.
We will also cover the difference between the team folder and team space configurations, as well as what happens when teams are migrated from team folders to team spaces.
Interacting with Team Content
Each Dropbox team may use one of two different configurations: team folders or team space. Different teams may also have different features enabled, which will affect how your app will need to operate. Team content is structured differently in each one, and that can affect how you use the Dropbox API to interact with team content.
In the team folder configuration, team folders are mounted in member folders:
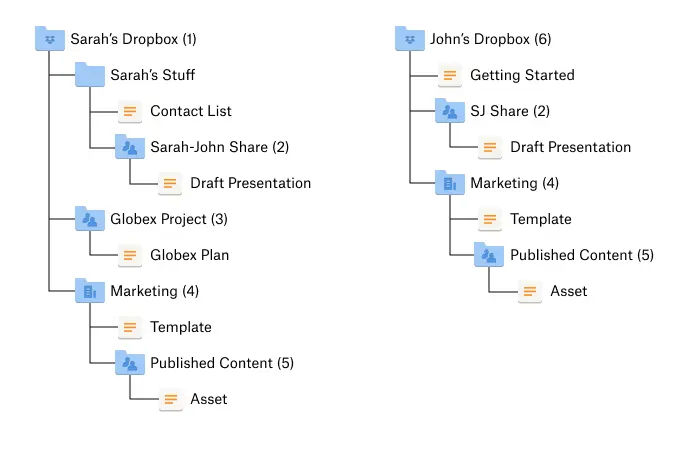
Team Folders model
Now, if their Dropbox team has the team space configuration, the team space will be structured as a root that contains all of the user’s folders, and any team folders they have access to. This is how Sarah and John's content would be organized:
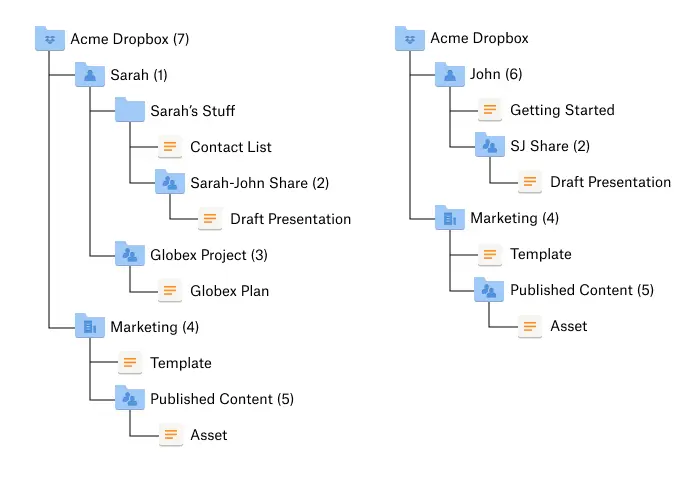
Team Spaces model
- The Acme Dropbox team root folder is a parent of both Sarah and John's home Dropbox Folders with namespace ID (7) if team_shared_dropbox is true; if it is false, then each team member account has its own root.
- "Marketing (4)" is a share under the Acme Dropbox team space, rather than a team folder mounted to member folders.
- The namespace IDs for existing mounted namespaces, such as shared folders, are unchanged.
- Teams using the team folders configuration may be migrated to use the team space configuration, resulting in team folders being moved to the team space.
API Call Roots
When you issue calls with the Dropbox API, the API calls that reference a specific file’s path are limited to the permissions authorized by the access token and relative to the namespace that the call is rooted to.
For an application with App Folder access, the call is rooted to an app folder within the team member’s folder. For an application with Full Dropbox access, the call is rooted to the team member’s folder by default.
In the example image above, if a Full Dropbox app authorized by John executed /files/list_folder it would be relative to namespace (6), and allow traversal content within that namespace. If John’s team uses the team folders configuration, this would include the Marketing team folder (4) he has access to. If the team uses the team space configuration, this would not include the Marketing folder (4) within the team space, as it is rooted to the team space.
Thus if a caller wished to access the team space, they would need to root their calls to the team space for the account. This would allow the app to access content accessible to and authorized by John, which would include the member folder and other folders he has access to in the team space. Data that John does not have permissions for, like Sarah’s folder (1), would not be visible or accessible.
To root a call to a specific namespace, a caller would need to identify that namespace and indicate it as a target root, as described in the sections below.
Identifying Root Namespaces
In order to access files on users’ namespaces, first you need to retrieve the namespace IDs from the API. This is done with the endpoint /users/get_current_account.
In the example above, let's call this endpoint with Sarah's user ID. If her team uses the team folders configuration, we'd get a response like this:
{
...
"root_info": {
".tag": "user",
"root_namespace_id": "1",
"home_namespace_id": "1",
}
}
Note that root_namespace_id and home_namespace_id are the same. The root of her Dropbox content is her home folder. Now if Sarah's team was on the team space configuration with has_team_shared_dropbox: true, the response would look like this:
{
...
"root_info": {
".tag": "team",
"root_namespace_id": "7",
"home_namespace_id": "1",
"home_path": "/Sarah"
}
}
In the team space configuration, the root namespace of Sarah’s account is different than her home namespace. Team content is under her root namespace (7). Her content and shared folders are mounted under namespace (1).
Using the Dropbox-API-Path-Root Header
The Dropbox-API-Path-Root header can be used to perform actions relative to a namespace. When using this header, all operations will be performed as if the specified namespace were the root. Response values like path_display and path_lower will also be relative to that root. This enables applications to access content in the team space, or to root to a particular namespace for syntactic convenience.
In this example, setting the Dropbox-API-Path-Root to access the root namespace with a token authorized to Sarah’s account with a team space would return content she has access to:
curl -X POST https://api.dropboxapi.com/2/files/list_folder \
--header 'Authorization: Bearer <token>' \
--header 'Dropbox-API-Path-Root: {".tag": "root", "root": "7"}' \
--header "Content-Type: application/json" \
--data '{"path":""}'
Response:
{
...,
"path_display": "/Sarah/Draft Presentation",
"path_lower" : "/sarah/draft presentation",
"name" : "Draft Presentation",
...,
"path_display": "/Marketing",
"path_lower" : "/marketing",
"name" : "Marketing",
...,
}
Remember, users with existing Dropbox accounts may join teams, causing their root folder to change (while their home folder stays the same). The path root header has alternate modes to help your application detect this change. See the Path Root Header Modes documentation for more information.
Using the Dropbox-API-Path-Root header is recommended as it works for both configurations. If you don’t set the Dropbox-API-Path-Root header, your app will not be able to access the contents of the team space (for any connected users with the team space configuration).
The official Dropbox SDKs also offer support for setting the Dropbox-API-Path-Root header. If you’re using an official Dropbox SDK, refer to the respective documentation for more information:
Managing Team Member Content
So far we have covered accessing content in company managed spaces with the User API. The Business API enables team administrators to both manage company owned spaces, as well as to manage the content of individual team members.
Applications that authorize team scopes receive a team-linked token for operating on Business API endpoints. These applications may also use any authorized User API calls to operate on behalf of members of the team by specifying the member ID in an HTTP header as long as the application has the team_data.member scope. Apps using the legacy permission model must instead select the access type of ‘Team member file access’.
Dropbox-API-Select-User
This header, when used with a team token, enables the application to issue calls on behalf of the specified team member. This enables applications to organize and act on content within users member folders.
Dropbox-API-Select-Admin
This header, when used with a team token, enables the application to issue calls as the specified team administrator.
Dropbox-API-Select-Admin is required when making modifications to team-managed team folders & team spaces using sharing API calls, as described in the next section.
When reading content and metadata, using Dropbox-API-Select-Admin enables the caller to see any team accessible content, without needing to determine which user on the team has view access. Using the /team/namespaces/list call to enumerate all team namespaces, then traversing them with /files/list_folder with the Dropbox-API-Select-Admin header enables an app to efficiently enumerate all team content.
Remember, files can be referred to in path arguments by their relative path, file ID, revision ID, or by a namespace-relative path. While most user-linked applications will prefer referring to files by ID or relative path, team linked applications looking to efficiently traverse all team-owned content will tend to find namespace-relative paths simpler.
Read more about how to use these headers in our Authentication Types Guide.
Managing Team Folders and Spaces
Team folders and spaces are the best way to organize and control content that belongs to the organization and not to specific users.
Determining the Team's Organizational Model
When reading or writing files to team folders or team spaces, the methodology is the same–specifying the Dropbox-API-Path-Root allows an application to access all authorized content in both models.
However, a team-linked application that wishes to create and modify these team-managed spaces may need to utilize different API calls to do so, and thus will first need to programmatically determine which model and features the team is using.
In the Dropbox web interface, it is easy to identify if your team is using the team space configuration: your team member folder is named after you.
Programmatically, there are a few ways of finding out which type of team you have:
- If using a team linked app, use the /team/features/get_values endpoint to check the has_distinct_member_homes boolean. If it's true, then the team uses the team space configuration.
- If using a user linked app, call /users/features/get_values and check the distinct_member_home boolean. If it's true, then the user’s team uses the team space configuration.
Additionally, there are a few ways to determining which features a team has:
- If using a team linked app, use the /team/features/get_values endpoint to check the has_team_shared_dropbox boolean. If it's true, then the team uses a single team space for all members of the team, and the contents can be managed directly in the team space using the /files endpoints. If it’s false, then the team’s team folders can be managed using the /team/team_folder endpoints.
- If using a user linked app, use the /users/features/get_values endpoint to check the team_shared_dropbox boolean. If it's true, then the user’s team uses a single team space for all members of the team, and the contents can be managed directly in the team space using the /files endpoints. If it’s false, then user-linked apps cannot create files or folders directly in a team space; files and folders may only be added inside team folders inside a team space.
If you need a Dropbox team to develop and test your integration and don’t already have one, refer to the “How do I test my application?” question under “Frequently Asked Questions” on the support page.
Managing Team Folders
Creating Team Folders
If the team has has_team_shared_dropbox: false and you need to create a new team folder, use the /team/team_folder/create endpoint. When you create a team folder programmatically, it doesn't give access to anyone automatically, not even to the Dropbox team administrators. You will need to add members to the team folder and configure its security settings after the folder has been created.
If the team has_team_shared_dropbox: true, and you try to issue the /team/team_folder/create call, you're going to get an error message. We cover that scenario in the next section under “Managing Team Spaces”.
Managing Team Folder Access
Since team folders are managed by Dropbox team administrators only, you will need to specify the admin ID that will be executing these operations via the Dropbox-API-Select-Admin header.
Each team folder can have different security settings. For example, you may want to create a team folder with content that can be shared externally and another team folder with content that is sensitive and should be internal only. This can be achieved by configuring the shared folder policy.
Update Folder Policy
The team folder security settings can be configured using the endpoint /sharing/update_folder_policy. This is similar to what a Dropbox administrator can do with the settings of a shared folder. The UpdateFolderPolicyArg object allows you to configure parameters such as:
- member_policy: who can be invited to this folder (team members only or any Dropbox user)
- acl_update_policy: who can invite/remove people to this folder (owner only or any member with editor access to the folder)
- viewer_info_policy: if Viewer Info is enabled or disabled
- shared_link_policy: who can view shared links on this folder (only team members or anyone)
For the full list of parameters please consult the HTTP endpoint documentation.
Adding people to team folders
The recommended access control practice is to use groups to assign permissions to team folders. This makes the security administration and maintenance a lot easier. In fact with team folders you cannot add individual users directly to the root folder; you are required to use groups. You can create groups via the API, add people, and then assign these groups to folders. Check the Team Administration Guide for information on how to manage groups.
To add a group to a team folder use the /sharing/add_folder_member endpoint, the .tag parameter dropbox_id and the group’s unique identifier as the value for dropbox_id.
Restricted access control lists
When you apply permissions to a team folder, by default all the folders in the hierarchy under that folder inherit the same permissions. But you can disable the inheritance and nest folders inside of a team folder or team space with different levels of permissions. Subfolders can have more restricted permissions than their parent folders.
To disable the inheritance, follow these guidelines:
- Restrict access to a new shared folder by calling /sharing/share_folder with access_inheritance set to no_inherit.
- Note that /sharing/share_folder will create a new folder if given a path that does not exist.
- Restrict access to an existing folder by calling /sharing/set_access_inheritance with access_inheritance set to no_inherit.
Once the inheritance is disabled, set your desired level of access with /sharing/add_folder_member.
Restoring inheritance:
- Calling /sharing/set_access_inheritance with access_inheritance set to inherit will restore all permissions inherited from the parent folder.
Deleting Team Folders
Because team folders contain information that is accessed by many people, you cannot just outright delete them. You need to first archive the team folder (/team/team_folder/archive), which makes it unavailable for users, and then permanently delete it (/team/team_folder/permanently_delete). Note that not even Dropbox Support can revert a permanent deletion. Archived folders can always be unarchived by using /team/team_folder/activate.
Managing Team Spaces
On teams with has_team_shared_dropbox: false, you have to use the Business API to create and set up team folders. But on teams with has_team_shared_dropbox: true, you use the same User API calls to work both with shared folders that are mounted under a user-owned namespace (also known as member folder), and with folders that are mounted in the team space namespace.
Attempting to use the team_folder/activate, team_folder/archive, team_folder/create, team_folder/permanently_delete, or team_folder/rename endpoints for a team with has_team_shared_dropbox: true will fail with either a team_shared_dropbox_error/disallowed or invalid_account_type/feature error. Calling team_folder/get_info and team_folder/list for a team with has_team_shared_dropbox: true will only return information about the team space.
For team-linked apps that need to access the team space, you’ll need both Dropbox-API-Path-Root and Dropbox-API-Select-Admin headers. If you don’t specify the Dropbox-API-Path-Root header, you won’t see team space content. Instead, you’ll be rooted to the user’s member folder rather than the team space.
Creating folders on Team Spaces
To create a folder for under the team space for a team with has_team_shared_dropbox: true you can use the /sharing/share_folder endpoint. When you use this endpoint and the folder doesn't exist yet, it will be created.
A few important things to note here when using it in the team space configuration:
- The Dropbox-API-Path-Root header can use one of the syntaxes described above in the Dropbox-API-Path-Root Header Modes section, to set this to the root namespace.
- The Dropbox-API-Select-Admin is the member ID of an admin, with the syntax dbmid:xxxxx
- The path parameter defines the name of the folder to be shared/created. It always starts with a slash ('/') character.
FAQ
- What happens when a team is migrated from the team folders configuration to the team space configuration?
- When a team is migrated from team folders tothe team space configuration, the team folders will be moved from the member folders to the new team space. You can find more information in this help center article.
- When a team is migrated from team folders tothe team space configuration, the team folders will be moved from the member folders to the new team space. You can find more information in this help center article.
- When happens if an app isn’t updated to support accessing the team space and a team is migrated to use the team space configuration?
- When a team is migrated to use the team space configuration, team folders are moved to the team space. By default, without setting the Dropbox-API-Path-Root header, API calls will operate in the member folder and will no longer return those team folders. If an app is relying on absolute paths to team folders in the member folders and doesn’t set the Dropbox-API-Path-Root header, those team folders will no longer be found at those paths when the team is migrated, causing those API calls to fail.
- Does a team’s namespace IDs change during the migration to the team space configuration?
- Existing team folders and shared folders keep their existing namespace IDs through the migration. Team members receive a new namespace ID for their root namespace.
- How can I check which configuration my own team currently uses?
- You can manually check which configuration your team uses via the Dropbox web site. Refer to this help center article for instructions on how to do so. To programmatically check which configuration a team is currently using via the API, refer to the "Determining the Team's Organizational Model” section above.
- How can I list the contents of the team space programmatically?
- To list the contents of the team space the user has access to, use /files/list_folder with the Dropbox-API-Path-Root header set to account’s root namespace ID as root and set the path to "". Refer to the “Using the Dropbox-API-Path-Root Header” section above for more information.
Additional Resources
- [Developer Blog Post] Manage team sharing in your Dropbox app
- [Developer Blog Post] Implement restrictive access using the Dropbox API
- [Developer Guide] Team Administration Guide